Using ChatGPT to Help With Micro:bit Coding
Tips and tricks for helping improve your code!
Written By: Marcus Schappi
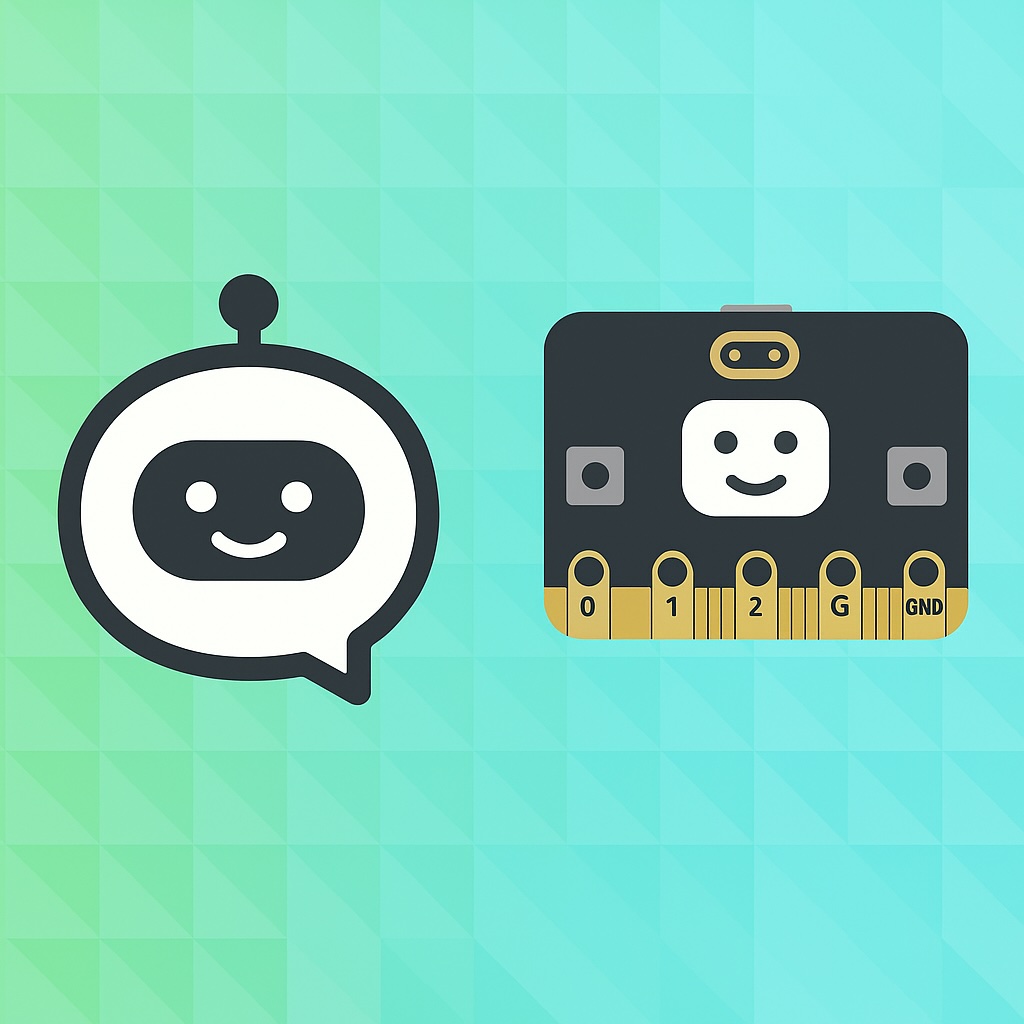

Difficulty
Easy

Steps
7
ChatGPT is like having a coding mentor, creative partner, and explainer all in one. Whether you're learning to program for the first time or building a custom classroom project, ChatGPT can help you:
- Understand how Micro:bit code works (in Python or blocks),
- Get working code examples quickly,
- Modify and troubleshoot your ideas,
- Explore new and exciting project ideas tailored to your level.
Begin by asking ChatGPT something specific.
Here are examples:
Here are examples:
- Simple:
“How do I make an LED blink on the Micro:bit?” - Intermediate:
“Can you help me write code for a Micro:bit compass?” - Project-Based:
“I want to make a Micro:bit pet that reacts to button presses. Can you help me start?”
💡 Tip: Mention whether you're using MakeCode (blocks) or MicroPython, so ChatGPT can give you the right type of code.
from microbit import * while True: if button_a.is_pressed(): display.show(Image.HEART) else: display.clear()
ChatGPT can provide:
- MicroPython Code for use at python.microbit.org
- MakeCode Instructions describing how to drag blocks and configure them at makecode.microbit.org
🟩 Example (MakeCode):
"Create a forever loop. Inside it, check if button A is pressed, then show a heart icon."
🟩 MakeCode Blocks Description:
"Create a forever loop. Inside it, check if button A is pressed, then show a heart icon."
🟩 MakeCode Blocks Description:
- Use a
forever
block. - Inside it, use an
if
block to checkbutton A is pressed
. - If true, use
show icon
→ heart. - Else, use
clear screen
.
from microbit import * while True: temp = temperature() display.scroll(str(temp)) sleep(2000)
Copy the code into your online editor or drag blocks into MakeCode.
Take the code and run it in:
Take the code and run it in:
- python.microbit.org for MicroPython
- makecode.microbit.org for block coding
Press "Download" and flash it to your Micro:bit.
See it in action and troubleshoot as needed!
from microbit import * while True: if button_b.is_pressed(): display.show(Image.HAPPY) else: display.clear()
Don’t stop at a basic version. Try:
- “Can you make it show a smiley face when I press button B?”
- “Add a temperature display using the onboard sensor.”
- “Make it beep when the accelerometer detects a shake.”
ChatGPT will help modify the code accordingly.
sleep(2000)
If you're unsure what something means, ask!
“What does sleep(2000)
do?”
ChatGPT will explain:
📘 "It pauses the program for 2000 milliseconds (2 seconds)."
Or ask:
“What’s the difference betweenbutton_a.was_pressed()
andbutton_a.is_pressed()
?”
When creating our "Whale of a Time" game, we were editing both code and hardware. Sometimes things didn't work as expected.
You can describe the problem to ChatGPT and it will help debug the problem.
eg. "it seems when the game is finished running, pressing the button doesn't reset it and start again - nothing happens"
and ChatGPT's response was -
"Thanks for flagging that — you're right. The issue lies in how the code runs the
You can describe the problem to ChatGPT and it will help debug the problem.
eg. "it seems when the game is finished running, pressing the button doesn't reset it and start again - nothing happens"
and ChatGPT's response was -
"Thanks for flagging that — you're right. The issue lies in how the code runs the
startGame()
function as a blocking loop(due to the countdown), which prevents the basic.forever
loop from detecting button presses again until it completes — and it doesn’t loop back properly for another run.Here’s how to fix it:...."
for (let y = 0; y < 5; y++) { for (let x = 0; x < 5; x++) { led.plot(x, y) basic.pause(1000) } }
Just because your code is working, doesn't mean it can't be improved!
We wanted a countdown in makecode that gave the player 25 seconds to complete the game.
My 7 year old daughter did the code by using blocks and would add one light to the matrix every second.
The code was VERY long - 215 lines!. Giving the raw code to ChatGPT it came back and made the entire code 6 lines and gave an explanation how it did it!
How it works:
We wanted a countdown in makecode that gave the player 25 seconds to complete the game.
My 7 year old daughter did the code by using blocks and would add one light to the matrix every second.
The code was VERY long - 215 lines!. Giving the raw code to ChatGPT it came back and made the entire code 6 lines and gave an explanation how it did it!
How it works:
- It starts at the top-left LED
(0, 0)
and moves row by row. - For each LED position, it uses
led.plot(x, y)
to turn it on. - It pauses for 1000 ms (1 second) after each LED is turned on, just like in your original code.
Output:
This version will light up one LED at a time from left to right, top to bottom, filling the entire 5×5 LED grid exactly as your long version did.